Chapter 8 The Basics
Section 8.1 Basic Syntax, Values and Operators
Subsection 8.1.1 Syntax
In Racket, we wrote and used a lot of functions. In NetLogo, we will use the word procedure instead. A procedure is similar in concept to a function. One big difference is that all our functions in Racket returned values (this does not have to be true, it is just how we used Racket). In NetLogo, it is much more common to have procedures that do not return values (in fact, many of them will result in visual changes in the NetLogo world instead.
We often talk about using commands in NetLogo. What we usually mean by this are built in procedures provided by NetLogo. Official NetLogo documentation may also refer to these procedures as primitives 1 . There are many NetLogo primitives, and we will not be able to learn them all in this class.
To run a command in NetLogo, we can use its name: If the command takes arguments, we separate them with spaces:
clear-all
create-turtles 10
All whitespace (space, newline, tab) is equivalent in NetLogo, so this: is equivalent to:
clear-all create-turtles 10
clear-all
create-turtles 10
Even though the two programs above are valid in NetLogo, let’s not forget about the utility of good programming style. The second version is much clearer than the first, putting each separate command on its own line.
Of course, the hallmark of Racket syntax is its use of prefix notation. NetLogo does not have such a strict adherence to doctrine. Some commands use prefix notation, others use infix (operator goes in-between the operands). This is something we will just need to keep track of as we learn new commands.
Subsection 8.1.2 Numbers and Arithmetic Operations
Unlike Racket, numbers in NetLogo are either going to be integer or floating point values. NetLogo does not have built in support for fractions, complex numbers, etc. We do use the same symbols for the basic arithmetic operators:
+ - * /
in addition, there are two more basic arithmetic operators:^
: Exponent operator.2 ^ 4
mod
: Modulus operator.20 mod 3
. This is similar to remainder, and works exactly like remainder if both operands are positive. Technically,a mod b
is \(a - (floor (a / b)) * b\)
Note that all arithmetic operators are infix, which is what we re used to from regular math. NetLogo does require spaces between operators and operands.
11 + 51
is valid while 11+51
is not.While not strictly an arithmetic operator, we will find that the ability to generate random values comes up quite often.
random n
: Return a random integer.- If
n
is positive, the value returned will be in the range [0, n) - If
n
is negative, the value returned will be in the range (-n, 0]
Subsection 8.1.3 Boolean Values, Comparison Operators, Boolean Operators
Boolean values in NetLogo are represented by the literals
true
and false
. The comparison operators are the same: = > < >= <=
. Like the arithmetic operators, theses are also infix. There is one additional operator !=
, which checks if two values are not equal.We also have our boolean operator friends,
and or not
. and
and or
are both infix operators, while not
is prefix. NetLogo also provides us with xor
, which is exclusive or. xor
is an infix operator that returns true
only when one of the operands is true
and returns false
in all other cases.Section 8.2 Units
Subsection 8.2.1 Colors
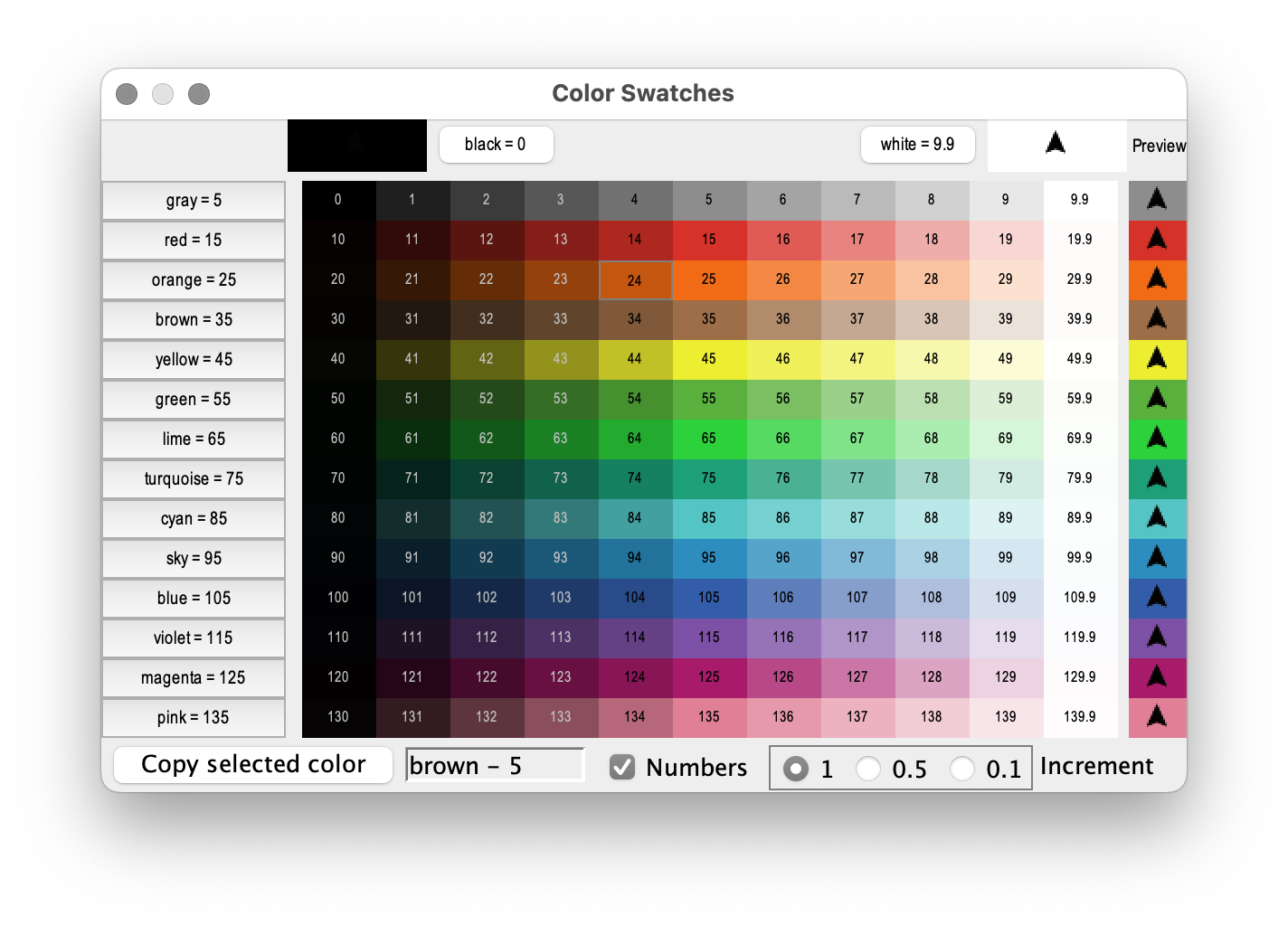
The image above is the NetLogo Color Swatches tool (accessible via the tools menu in NetLogo). We can learn all we need to know about NetLogo colors from this image. Here are the big ideas:
- A color is a numeric value in the range [0, 140).
- There are 16 named colors. You can see 14 of them on the left side of the window (
gray red orange
...) and thenblack
andwhite
. black
has the value of0
.white
has the value of9.9
.- All the other named colors are values that end in
5
. The color names themselves are jsut defined constants in NetLogo, so if you uselime
in your code, it is the same as65
. - Each row is a gradient of a named color, starting with a multiple of
10
which is almost black and ending at a value that ends in9.9
which is almost white. - While the chart is mostly integer based, you can use non integer values to get in-between shades.
Subsection 8.2.2 Sizes & Length
The basic unit of measurement in NetLogo is the side length of a patch (patches are always square). This shows up in a few different ways, especially for turtles. Turtle motion will always be based on this measurement. So a turtle moving forward 1 unit would move forward by a patch-length. Turtle size is also proportional to this patch-length unit. Size 1 means the longest dimension of the turtle (this will depend on its shape) will be at most 1 patch-length.
Section 8.3 Properties
Now we can finally do something with the patch and turtle properties we saw before. Some specific examples:
set
is a versatile NetLogo command that allows us to change the value of any variable, which includes all turtle and patch properties. Generally, set
works as follows: set VARIABLE VALUE
set pcolor pink
set size 3
set shape "turtle"
Now we need to start being careful about context. In general, you can only use
set
on a property that exists in the provided context:- In observer context, you cannot use
set
to directly change any properties, because the observer has no properties. - In patch context, you can use
set
to directly modify any patch property (the ones that start withp
). But you cannot directly modify turtle properties. - In turtle context, you can use
set
to directly modify any turtle property. You can also useset
to directly modify the properties of the patch the turtle is currently on top of.
Section 8.4 Command Starter Pack!
Now that we’ve got a handle on the basics, lets get into some of the many, many, many 2 built in NetLogo commands. Since context is key, we’ll separate them out by context. Some of the commands have shortened versions of names (like
clear-all
and ca
), when these shortened versions exist, they will be included in the examples.Subsection 8.4.1 Turtle Context
The following commands only work in turtle context. They largely deal with turtle movement, since that is what makes turtles special.
forward d
: Have a turtle move in the direction it is facingd
steps. Ifd
is negative, the turtle will move backward.forward 10
fd 2
backward d
: Have a turtle move away from the direction it is facingd
steps. Ifd
is negative, the turtle will move forward.backward 5
bk 7
right a
:Have a turtle turn to the righta
degrees. Ifa
is negative, the turtle will turn left.right 45
rt 236
left a
: Have a turtle turn to the lefta
degrees. Ifa
is negative, the turtle will turn right.left 45
lt 236
pen-down
: Instruct a turtle to start drawing. When a turtle’s pen is down, it will leave a trail whenever it moves. The line drawn will be the same color as the turtle. The line will display on top of the patches but below the turtles. Thepen-size
turtle property controls the width of the line.pd
is the shortened version.pen-up
: Instruct a turtle to stop drawing. This reverses thepd
command.pu
is the shortened version.stamp
: Instruct a turtle to leave an image of itself at its current position. The stamped image will be above the patches and below turtles.setxy x y
: Move the turtle to (x
,y
). This is essentially a faster way of doingset xcor x
andset ycor y
.
Subsection 8.4.2 Observer Context
clear-all
: Reset the entire NetLogo world. Upon runningclear-all
(orca
) the following will happen:- All turtles will be removed.
- All drawing (lines and stamps left by turtles) will be cleared.
- All the patches will be set back to black.
clear-drawing
: Clear all the lines and stamps left by turtles. Runningclear-drawing
(orcd
) will not have any effect on the turtles or patches.create-turtles n
: Createsn
turtles. Each turtle will be located at the origin, have a size of 1, be given a random heading, and a random color chosen from the 14 "primary" NetLogo colors (this excludes black and white). Optionally, you can provide turtle-specific commands inside[]
. These commands will be run by each turtle after they have all been created.create-turtles 5
crt 5 [pd fd 10]
create-ordered-turtles n
: Createsn
turtles. Each turtle will be located at the origin, have a size of 1. The headings of the turtles will be evenly spaced from 0 to 360. The colors will be assigned from the 14 primary NetLogo colors starting atgray
(5
), and cycling back again if more than 14 turtles are created. Likecrt
, you can provide turtle-specific commands inside[]
. These commands will be run by each turtle after they have all been created.create-ordered-turtles 5
cro 5 [pd fd 10]